UART
Function Description
Interrupt mode and DMA mode
- Features of UART
- High Speed UART (max baud rate 4MHz and DMA mode) and low speed UART (IO mode)
- UART (RS232 Standard) Serial Data Format
- Transmit and Receive Data FIFO
- Programmable Asynchronous Clock Support
- Auto Flow Control
- Programmable Receive Data FIFO Trigger Level
- DMA data moving support to save CPU loading
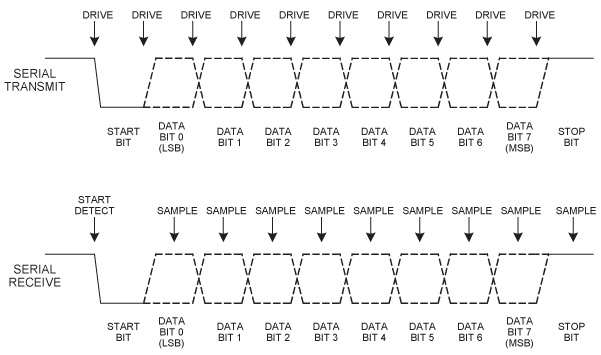
- Interrupt mode
/* send & recv one byte*/ serial_putc(sobj, *(pstr+i)); serial_getc(&sobj); /* send & recv target length data use interrupt mode */ serial_send_stream(sobj, pstr, _strlen(pstr)); serial_recv_stream(&sobj, rx_buf, 8);
- DMA mode
/* send & recv target length data use DMA mode */ serial_send_stream_dma(sobj, pstr, _strlen(pstr)); serial_recv_stream_dma(&sobj, rx_buf, 13);
- Interrupt + DMA mode for UART RX
void main(void) { char temp[128]; char temp2[8]; int actual_len=0; rx_semaphore = xSemaphoreCreateCounting(0xFFFFFFFF, 0); DiagPrintf("===Receive start===\r\n"); int32_t received; while(1) { serial_init(&serial, PA_7, PA_6); serial_baud(&serial, 115200); serial_format(&serial, 8, ParityEven, 1); serial_set_flow_control(&serial, FlowControlRTSCTS, 0, 0); /*recv data use interrupt mode */ received = serial_recv_blocked(&serial, temp2, 1, 500); if(received>0) { memcpy(temp+actual_len,temp2,received); actual_len += received; while(1) { /*recv data use DMA mode */ received = uart_recv(temp2,1,30); if(received>0) { memcpy(temp+actual_len,temp2,received); actual_len += received; if(actual_len==16) { for(int i=0;i<actual_len;i++) { DiagPrintf("%02x ", temp[i]); } DiagPrintf("\r\n"); actual_len = 0; break; } } } } serial_free(&serial); } for(;;){/*halt*/} }
Copyrights ©瑞晟微电子(苏州)有限公司 2021. All rights reserved. Terms of Use